Downloading features
After uploading data and editing it, we want to let our users download the result. To do this, we'll serialize our feature data as GeoJSON and create an <a>
element with a download
attribute that triggers the browser's file save dialog. At the same time, we'll add a button to the map that let's users clear existing features and start over.
First, we need a bit of markup to represent the buttons. Add the following elements after the map-container
in your index.html
:
<div id="tools">
<a id="clear">Clear</a>
<a id="download" download="features.json">Download</a>
</div>
Now we need some CSS to make the buttons look right. Add something like this to the <style>
element in index.html
:
#tools {
position: absolute;
top: 1rem;
right: 1rem;
}
#tools a {
display: inline-block;
padding: 0.5rem;
background: white;
cursor: pointer;
}
Clearing features is the easier part, so we'll do that first. The vector source has a source.clear()
method. We want clicks on the "Clear" button to call that method, so we'll add a listener for click
in our main.js
:
const clear = document.getElementById('clear');
clear.addEventListener('click', function () {
source.clear();
});
To serialize our feature data for download, we'll use a GeoJSON
format. Since we want the "Download" button to work at any time during editing, we'll serialize features on every change
event from the source and construct a data URI for the anchor element's href
attribute:
const format = new GeoJSON({featureProjection: 'EPSG:3857'});
const download = document.getElementById('download');
source.on('change', function () {
const features = source.getFeatures();
const json = format.writeFeatures(features);
download.href =
'data:application/json;charset=utf-8,' + encodeURIComponent(json);
});
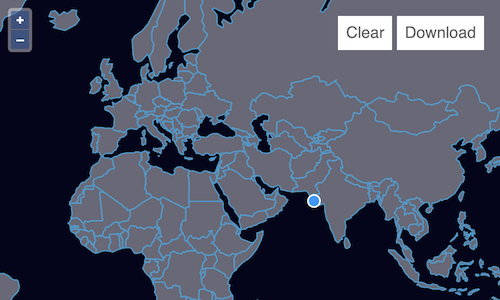