Rendering GeoJSON
Before getting into editing, we'll take a look at basic feature rendering with a vector source and layer. The workshop includes a countries.json
GeoJSON file in the data
directory. We'll start by just loading that data up and rendering it on a map.
First, edit your index.html
in order to add a dark background to the map:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>OpenLayers</title>
<style>
@import "node_modules/ol/ol.css";
</style>
<style>
html, body, #map-container {
margin: 0;
height: 100%;
width: 100%;
font-family: sans-serif;
background-color: #04041b;
}
</style>
</head>
<body>
<div id="map-container"></div>
<script src="./main.js" type="module"></script>
</body>
</html>
Now we'll import the three important ingredients for working with vector data:
- a format for reading and writing serialized data (GeoJSON in this case)
- a vector source for fetching the data and managing a spatial index of features
- a vector layer for rendering the features on the map
Update your main.js
to load and render a local file containing GeoJSON features:
import GeoJSON from 'ol/format/GeoJSON';
import Map from 'ol/Map';
import VectorLayer from 'ol/layer/Vector';
import VectorSource from 'ol/source/Vector';
import View from 'ol/View';
// assign to a variable named `map` for later use
const map = new Map({
target: 'map-container',
layers: [
new VectorLayer({
source: new VectorSource({
format: new GeoJSON(),
url: './data/countries.json',
}),
}),
],
view: new View({
center: [0, 0],
zoom: 2,
}),
});
You should now be able to see a map with country borders at http://localhost:5173/.
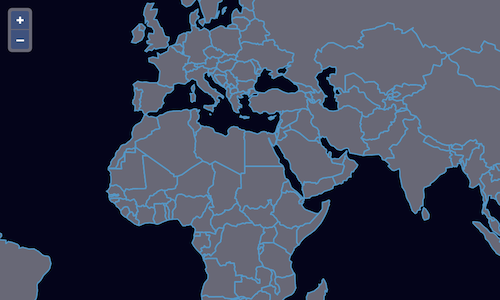
Since we'll be reloading the page a lot, it would be nice if the map stayed where we left it in a reload. We can bring in the Link
interaction to make this work.
In our main.js
we'll import the new interaction:
import Link from 'ol/interaction/Link';
And now we can add a new link interaction to our map:
map.addInteraction(new Link());
Now you should see that page reloads keep the map view stable. And the back button works as you might expect.