The VectorTile layer
We now know how to load tiled images, and we have seen different ways to load and render vector data. But what if we could have tiles that are fast to transfer to the browser, and can be styled on the fly? Well, this is what vector tiles were made for. OpenLayers supports vector tiles through the VectorTile
layer.
A world map rendered from vector data
We'll start with the same markup in index.html
as in the Basics exercise.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>OpenLayers</title>
<style>
@import "node_modules/ol/ol.css";
</style>
<style>
html, body, #map-container {
margin: 0;
height: 100%;
width: 100%;
font-family: sans-serif;
}
</style>
</head>
<body>
<div id="map-container"></div>
<script src="./main.js" type="module"></script>
</body>
</html>
As usual, we save index.html
in the root of our workshop folder.
For the application, we'll start with a fresh main.js
in the root of the workshop folder, and add the required imports:
import MVT from 'ol/format/MVT';
import VectorTileLayer from 'ol/layer/VectorTile';
import VectorTileSource from 'ol/source/VectorTile';
import {Map, View} from 'ol';
import {fromLonLat} from 'ol/proj';
The data source we are going to use is a simple map of the countries of the world from Natural Earth data, served as vector tiles by GeoServer.
The map setup we're going to create here is the same that we have used in previous exercises:
const map = new Map({
target: 'map-container',
view: new View({
center: fromLonLat([0, 0]),
zoom: 2,
}),
});
The layer type we are going to use this time is a VectorTileLayer
, with a VectorTileSource
:
const layer = new VectorTileLayer({
source: new VectorTileSource({
format: new MVT(),
url:
'https://ahocevar.com/geoserver/gwc/service/tms/1.0.0/' +
'ne:ne_10m_admin_0_countries@EPSG%3A900913@pbf/{z}/{x}/{-y}.pbf',
maxZoom: 14,
}),
});
map.addLayer(layer);
Our data source provides only zoom levels 0
to 14
, so we need to pass this information to the source. Vector tile layers are usually optimized for a tile size of 512 pixels, which is also the default for the VectorTile source's tile grid. The data provider requires us to display some attributions
, which we are adding to the source configuration as well.
As you can see, a VectorTileSource
is configured with a format
and a url
, just like a VectorSource
. The MVT
format parses Mapbox Vector Tiles. Like with raster tiles, the tile data is accessed by zoom level and x and y coordinates of the tile. Therefore, the URL includes a {z}
placeholder for the zoom level, and {x}
and {y}
placeholders for the tile coordinates.
The working example at http://localhost:5173/ shows an unstyled vector tile map like this:
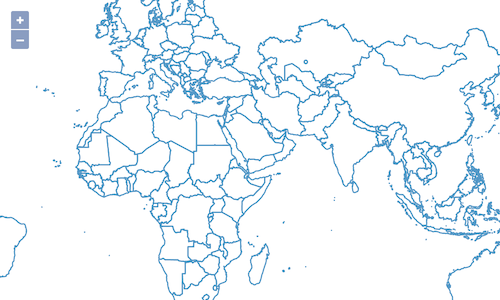