Visualization chooser (continued)
The final missing piece of our COG explorer is to make it so the user can choose the imagery source. While this section is already getting quite long, you may be able to tell that there is not that much more work involved in adding an image chooser. Let's do it!
The first thing we need to do is come up with a list of images that should be displayed. For each image, we want to display a name
to show the user in a <select>
element, and we need to have a base
for the GeoTIFF URL. So we'll want to add something like this to your main.js
:
const images = [
{
name: 'Buenos Aires',
base: 'https://sentinel-cogs.s3.us-west-2.amazonaws.com/sentinel-s2-l2a-cogs/21/H/UB/2021/9/S2B_21HUB_20210915_0_L2A',
},
{
name: 'Minneapolis',
base: 'https://sentinel-cogs.s3.us-west-2.amazonaws.com/sentinel-s2-l2a-cogs/15/T/WK/2021/9/S2B_15TWK_20210918_0_L2A',
},
{
name: 'Cape Town',
base: 'https://sentinel-cogs.s3.us-west-2.amazonaws.com/sentinel-s2-l2a-cogs/34/H/BH/2021/9/S2B_34HBH_20210922_0_L2A',
},
];
Next, we'll add another <select>
element to let the user choose the image. In your index.html
, adjust the controls to look like this:
<div id="controls">
<select id="image"></select>
<select id="visualization"></select>
</div>
Back to the JavaScript, we need to populate that <select>
element with one <option>
for each imagery source. In your main.js
add the following:
const imageSelector = document.getElementById('image');
images.forEach((image) => {
const option = document.createElement('option');
option.textContent = image.name;
imageSelector.appendChild(option);
});
Now we just need to make a minor adjustment to the function that updates our visualization so it grabs the base
URL from the selected imagery source. In main.js
edit the updateVisualization
function and surrounding lines so it looks something like this:
let previousBase;
function updateVisualization() {
const visualization = visualizations[visualizationSelector.selectedIndex];
const base = images[imageSelector.selectedIndex].base;
const newBase = base !== previousBase;
previousBase = base;
const layer = createLayer(base, visualization);
map.setLayers([layer]);
if (newBase) {
map.setView(layer.getSource().getView());
}
}
visualizationSelector.addEventListener('change', updateVisualization);
imageSelector.addEventListener('change', updateVisualization);
updateVisualization();
Note that we also only now update the view if the user has selected a new imagery source. This is nicer than zooming out to the full extent whenever a new visualization type is selected.
Reload http://localhost:5173/. Boom!
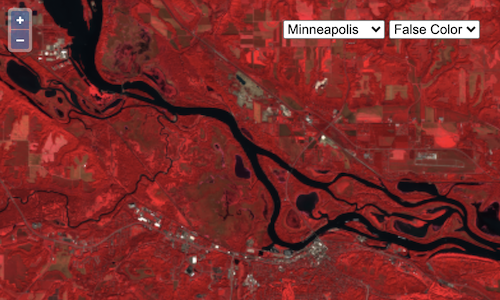